Variables are the identifier of the memory location, which used to save data temporary for later use in the program.
Variable Definition in Java
Syntax:
type variable_name;
type variable_name, variable_name, variable_name;
Example:
/* variable definition and initialization */
int width, height=5;
char letter='C';
float age, area;
double d;
/* actual initialization */
width = 10;
age = 26.5;
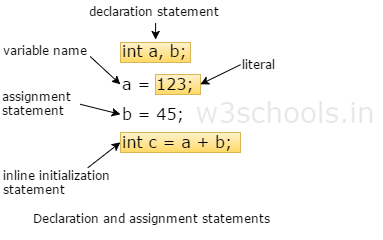
Variable name need to be chosen by the programmer in a meaningful way so that it reflects what it is representing within a program.
Rules of Declaring variables in Java
Types of Variables in Java
There are three types of variables in Java:
Local variables
A variable that is declared within the method that is called local variables.
Instance variables
A non static variable that is declared within the class but not in the method is called instance variable.
Class/Static variables
A variable that is declared with static keyword in a class but not in the method is called static or class variable.
Example:
class A {
int amount = 100; //instance variable
static int pin = 2315; //static variable
public static void main(String[] args) {
int age = 35; //local variable
}
}
Example:
public class Example { int salary; // Instance Variable public void show() { int value = 2; // Local variable value = value + 10; System.out.println("The Value is : " + value); salary = 10000; System.out.println("The salary is : " + salary); } public static void main(String args[]) { Example eg = new Example(); eg.show(); } }
0 comments:
Post a Comment